Qt提供了大量基本UI组件类,可以满足我们开发需求,下来列举一些常用组件。
QLabel类 #
QLabel类可以显示文字,图片等内容。
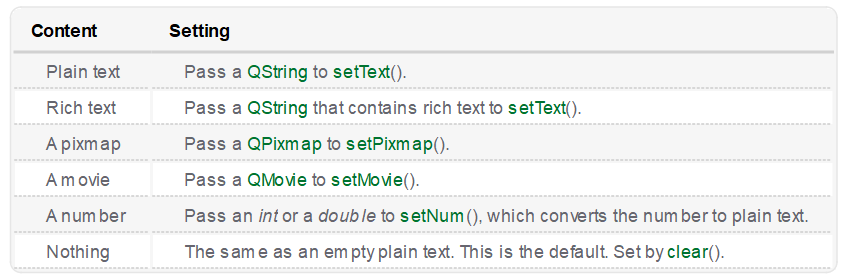
演示代码。
#include <QApplication> #include <QLabel> int main(int argc, char *argv[]) { QApplication a(argc, argv); QLabel label("hello world"); label.show(); return a.exec(); }
运行结果。
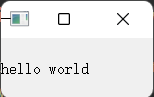
QPushButton类 #
QPushButton类提供了一个按钮的功能,常用来接受用户鼠标点击事件。
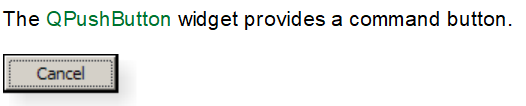
演示代码。
#include <QApplication> #include <QPushButton> int main(int argc, char *argv[]) { QApplication a(argc, argv); QPushButton button("OK"); button.show(); return a.exec(); }
运行结果。
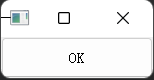
QLineEdit类 #
QLineEdit类是一个单行的编辑框,可用来接收少量文本输入,例如账号密码等。
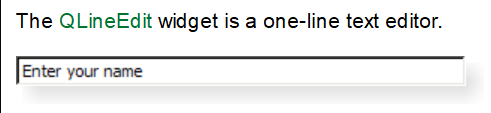
演示代码。
#include <QApplication> #include <QLineEdit> int main(int argc, char *argv[]) { QApplication a(argc, argv); QLineEdit edit("hello"); edit.show(); return a.exec(); }
运行结果。
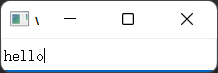
QGroupBox类和QRadioButton类 #
QGroupBox类可以将多个组件进行分组,有利于界面美观整洁。也可用于实现单选按钮的互斥。
演示代码。
#include <QApplication> #include <QGroupBox> #include <QRadioButton> #include <QVBoxLayout> #include <QObject> int main(int argc, char *argv[]) { QApplication a(argc, argv); QGroupBox *groupBox = new QGroupBox(QObject::tr("Exclusive Radio Buttons")); QRadioButton *radio1 = new QRadioButton(QObject::tr("&Radio button 1")); QRadioButton *radio2 = new QRadioButton(QObject::tr("R&adio button 2")); QRadioButton *radio3 = new QRadioButton(QObject::tr("Ra&dio button 3")); radio1->setChecked(true); QVBoxLayout *vbox = new QVBoxLayout; vbox->addWidget(radio1); vbox->addWidget(radio2); vbox->addWidget(radio3); vbox->addStretch(1); groupBox->setLayout(vbox); groupBox->setWindowTitle("https://dashima.net/"); groupBox->show(); return a.exec(); }
运行结果。三个单选框互斥,既就是只能选中一个。
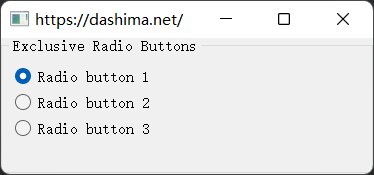
QProgressBar类和QTimer类 #
QProgressBar类提供一个进度条的功能。
QTimer提供一个定时器的功能,UI不显示。
演示代码。
#include <QApplication> #include <QProgressBar> #include <QTimer> #include <QObject> int main(int argc, char *argv[]) { QApplication a(argc, argv); QTimer timer; timer.setInterval(500); QProgressBar *bar= new QProgressBar(); bar->setRange(0, 100); bar->setValue(0); bar->setTextVisible(true); QObject::connect(&timer, &QTimer::timeout, [=](){ bar->setValue(bar->value()+1); }); bar->show(); timer.start(); return a.exec(); }
运行结果。
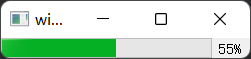
QSlider类和QLabel类 #
QSlider是一个滑块条,可供用户拖动调节参数。
演示代码。
#include <QApplication> #include <QSlider> #include <QLabel> #include <QHBoxLayout> #include <QObject> int main(int argc, char *argv[]) { QApplication a(argc, argv); QLabel label; QSlider slider; slider.setRange(0, 100); slider.setOrientation(Qt::Orientation::Horizontal); QObject::connect(&slider, SIGNAL(sliderMoved(int)), &label, SLOT(setNum(int))); QHBoxLayout hl; hl.addWidget(&slider); hl.addWidget(&label); QWidget widget; widget.setLayout(&hl); widget.show(); return a.exec(); }
运行结果。label内容随着滑块的改变而改变。
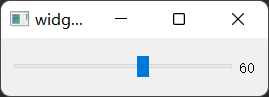
更多 #
我们可以在设计界面更直观的看到各种UI组件。
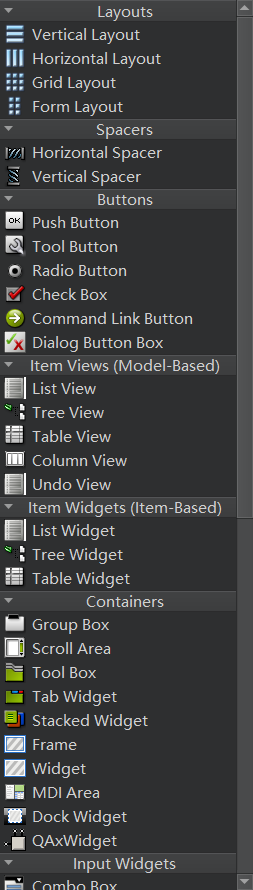
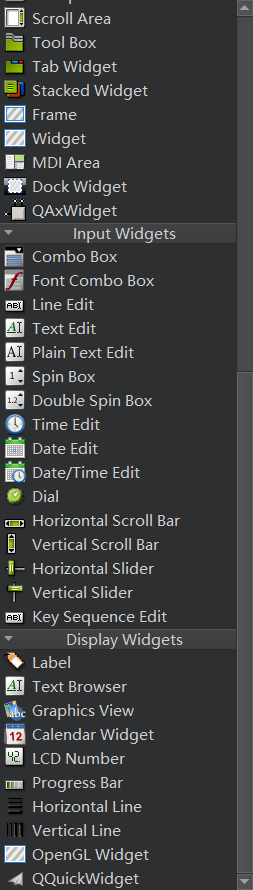
Qt库中所有类都是以大写的Q字母开头,可在Qt的帮助文档中搜索类名,查看更具体的介绍。
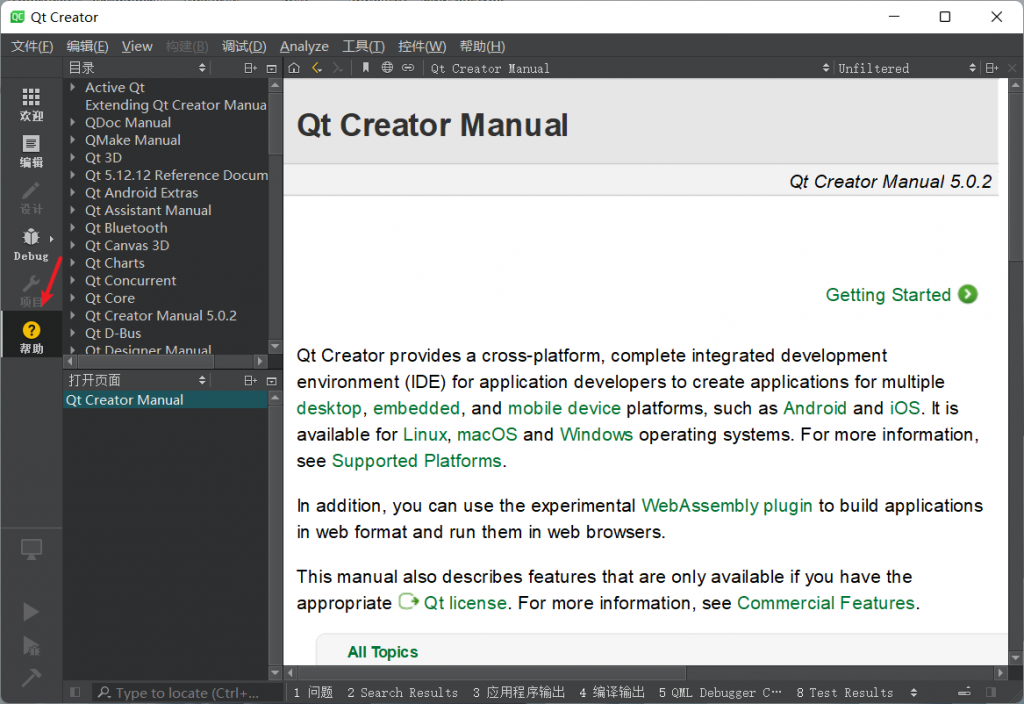
点击 帮助》索引 进行关键字检索。
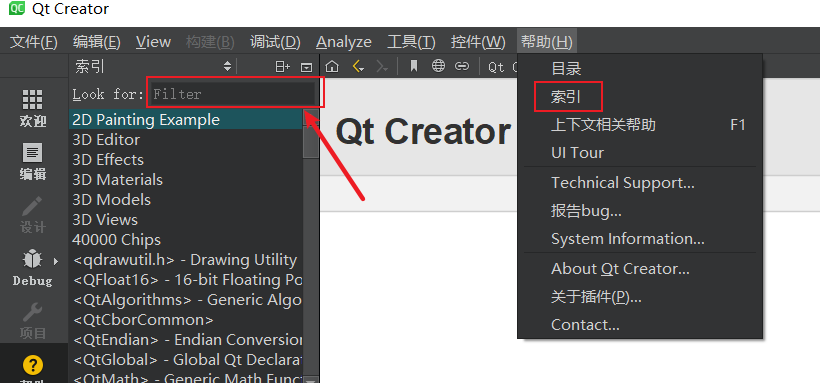
Qt中所有能在桌面显示的类,他们都继承自QWidget,非UI相关的类继承自QObject。
Qt官方在线文档: https://doc.qt.io/qt-5.15/